Introduction: A Vision towards addressing Waste Management Challenges using Smart Dustbins.
With growing urban population, arduino uno spec, efficient waste management is a critical challenge. The Smart Dustbin is a solution to this one based on innovation, that includes automatic opening of its lid when a user with waste approaches. For this project, I have created a Smart Dustbin (we don’t want it to be a bin in this case) using Arduino Nano with ultrasonic and IR sensors. Previous designs of smart dustbin were based on using ultrasonic sensors, but this project is made more efficient by using multiple sensors and being hands free.
The Objectives of Smart Dustbin Project
The central characteristic is to design a trash can that automatically opens its lid to a person or object to provide a cleaner and effortless experience. In this system an ultrasonic sensor is placed at the top of the dustbin lid to detect the presence of dustbin when cover is on and an IR sensor is added to provide functionality when the proximity cannot be detected due to minimal physical contact from user, as in the case of when user only moves a foot or touches dustbin with hand. The sensors communicate with an Arduino Nano, which controls two servo motors that open or close the dustbin lid.
Project Advantages
The Smart Dustbin is inspired by cleanliness campaign in India known as “Swachh Bharat Mission”. The purpose of this system is to provide a hand free way of using waste disposal into wet lab areas to promote a clean and eco friendly environment. It is designed using an Arduino Nano microcontroller board having a reputation of super small size and low power consumption. Incorporation of these ultrasonic and IR sensors guarantees efficient and contactless operation, as well as promoting a hygienic environment.
The Smart Dustbin components form the following key components of the Smart Dustbin project:
To build the Smart Dustbin using Arduino Nano, the following components are required:
Arduino Nano: Its the central microcontroller that controls the system.
Two Servo Motors (MG996R): To open and close the lid.
HC-SR04 Ultrasonic Sensor: For proximity detection.
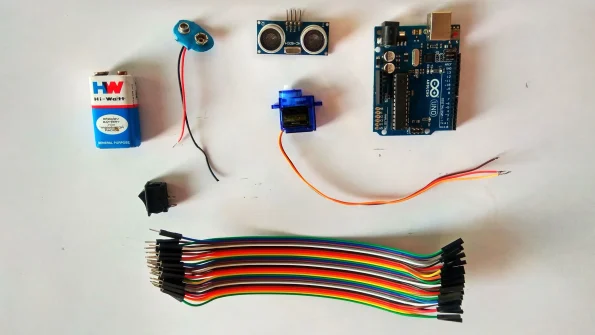
Generic IR Sensor: To detect minimal movement close to dustbin.
Two LEDs: Visual feedback should be provided for operations of the lid.
7.4V Li-ion Battery: Powers the entire system.
LM2596 DC-DC Buck Converter: It drops the voltage down from 12V to 8V and for power management purposes.
DC Socket and Switch: For easy on/off and charging.
Jumper Wires, Breadboard, or PCB: Also used for connections and wiring.
The working mechanism of Smart Dustbin
The control unit is the Arduino Nano which gets input from the ultrasonic and the IR sensors. Here’s a detailed breakdown of the smart dustbin’s working process:
Detecting Presence:
To monitor proximity, an HC-SR04 Ultrasonic Sensor is installed on top of the dustbin. It constantly emits sound waves, and calculates how long it takes them to bounce back. In this project, the preset range of the sensor is set to 40 cm to which whenever an object (e.g. a person’s hand or trash) enters the preset range the Arduino Nano is signaled to open the lid by rotating both servo motors in opposite directions.
Hands-Free Operation with IR Sensor:
Along with the ultrasonic sensor, a generic IR sensor is mounted at the bottom of the dustbin. The dustbin can open if a foot or another part of the body is nearby without physically touching the bin with this sensor. It will trigger and release the lid for any movement within its field.
Smooth Lid Closing:
After the trash is thrown away and the sensor stops recognizing an object, the Arduino Nano waits around two seconds to give commands to the servo motors to spin back and close the lid. This guarantees sleek and constant closure action, the preventative of sudden movements.
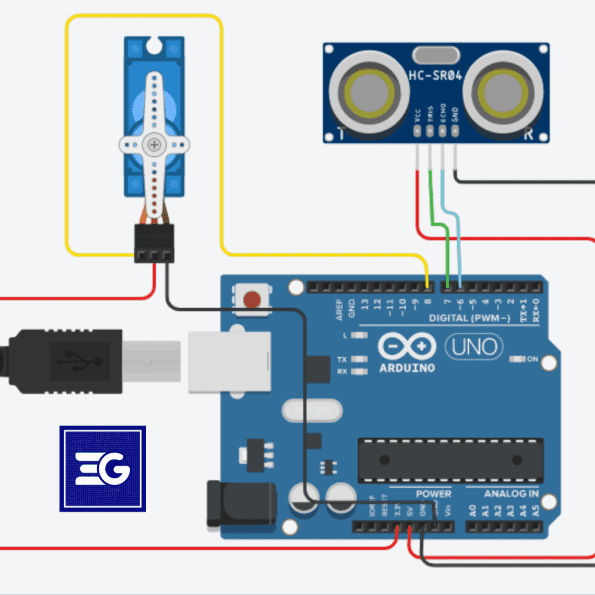
Arduino Uno Spec
A microcontroller board, the Arduino Nano is small, compact, and versatile. It consists of an ATmega328 microcontroller with enough power to easily run projects as small as this smart dustbin. Some of its key specifications include:
Operating Voltage: 5V
Input Voltage: 7-12V (recommended)
Digital I/O Pins: 14 (with 6 PWM outputs)
Analog Input Pins: 8
Flash Memory: Inside memory 32 KB (including 2 KB for the bootloader)
Clock Speed: 16 MHz
Its small form factor and many digital and analog pins make the Arduino Nano an obvious choice for such a project which involves multiple sensors and actuators as in this Smart Dustbin.
The ciruit diagram of the Smart Dustbin can be seen above.
The Smart Dustbin makes use of Arduino Nano in circuit design which is very simple because it is simply made up of Arduino Nano, ultrasonic sensor, IR sensor and two servo motors. The key considerations for the circuit include:
Feeding 7.4V Li-ion battery through a switch to Vin pin of Arduino Nano.
LM2596 buck converter has 8.1V setting the output voltage to regulate charging and power as efficiently as possible.
Connecting each of the servo motors to the correct PWM pins on the Arduino (Digital pins 9 and 5).
Ground wires of all of the components were linked to the GND pin of the Arduino Nano.
Design and Assembly
Furthermore, the lid open and close mechanism and ultrasonic sensor holder were designed with CAD tools such as Onshape. It enabled a precise and robust design for the components of the dustbin. All the parts are screw, nuts and hot glue secured to the dustbin body, so it won’t come loose and cause a short circuit.
Smart Dustbin is a very simple concept tool that operates on a relay.
However, in practice, when a hand or foot is brought close to the dustbin, the ultrasonic or IR sensor triggers, and a signal is sent to the Arduino Nano. To activate this, the dustbin lid opens as the servomotors are activated. Visual feedback during operation is given by indicator LEDs used by the system. The lid automatically closes after a short delay if the user moves their hand or foot away, giving a seamless, hands free waste disposal experience.
Smart Dustbin using Arduino: A Comprehensive Guide
In this project, we’ll build a “Smart Dustbin” using an Arduino, two sensors, and a couple of servo motors to automatically open and close the lid of the dustbin when an object is detected. The sensors employed for detecting objects are an ultrasonic sensor and an IR sensor. When a hand or foot comes within the detection range of either sensor, the Arduino processes the signals and activates two servo motors. These servos rotate in opposite directions to open the dustbin lid. Additionally, an indicator LED lights up to show that the bin is in use. Once the object moves out of range, after a delay of 2 seconds, the lid automatically closes.
Below is the code and an in-depth explanation of how each part works:
#include <Servo.h>
This line imports the Servo library, which is essential for controlling the servo motors. Servo motors allow us to precisely control the position of the dustbin lid by adjusting the angle at which it opens and closes.
const int trigPin = 2; // Trig pin of the ultrasonic sensor
const int echoPin = 3; // Echo pin of the ultrasonic sensor
const int irSensorPin = 4; // IR sensor pin
const int ledPin = 7; // LED pin
const int lightPin = 6; // Light pin
Here, constants are defined for the pin numbers that will be used to control various components. These pins correspond to specific physical pins on the Arduino board. The trigPin and echoPin are connected to the ultrasonic sensor, which helps in detecting the distance to an object. The irSensorPin is connected to the IR sensor for additional object detection. The ledPin and lightPin control the LED and a light indicator, respectively, giving visual feedback when the dustbin lid is opened or closed.
Servo servo1;
Servo servo2;
bool lidOpen = false; // Variable to track the lid status
unsigned long lastObjectTime = 0; // Timestamp to track when object was last detected
Here, we declare two servo objects, servo1 and servo2, which will control the two servos responsible for opening and closing the dustbin lid. The boolean variable lidOpen tracks the status of the lid (whether it is open or closed). The lastObjectTime variable stores the timestamp when an object was last detected by the sensors, allowing us to implement a delay mechanism for closing the lid after the object has moved away.
The Setup Function
void setup() {
servo1.attach(9);
servo2.attach(5);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(irSensorPin, INPUT);
pinMode(ledPin, OUTPUT);
pinMode(lightPin, OUTPUT);
servo1.write(90); // Initial position (lid closed)
servo2.write(90); // Initial position (lid closed)
delay(1000);
Serial.begin(9600);
}
In the setup() function, the servo motors are attached to pins 9 and 5 on the Arduino. These pins control the movement of the servos that open and close the dustbin lid. The ultrasonic sensor’s trigPin is configured as an output pin (to send signals), while the echoPin is set as an input pin (to receive signals). Similarly, the irSensorPin is set as an input to detect objects via the IR sensor. The ledPin and lightPin are configured as outputs to control the LED and light indicators.
To ensure the lid starts in a closed position, both servos are initialized to 90 degrees (a neutral position). A 1-second delay is added to stabilize the system during startup. Serial communication is also initialized with a baud rate of 9600, allowing us to print values for debugging purposes through the serial monitor.
The Main Loop
void loop() {
long duration;
int distance;
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration / 2) / 29.1;
Serial.println(distance);
In the main loop, the Arduino constantly monitors the distance between the sensor and any approaching object. The ultrasonic sensor works by sending out a sound wave and measuring the time it takes for the wave to bounce back from an object. By calculating the duration of the echo, the distance is determined. The formula (duration / 2) / 29.1 converts the time into centimeters.
The calculated distance is printed to the serial monitor for debugging purposes, allowing us to see how close an object is to the sensor in real-time.
int irSensorValue = digitalRead(irSensorPin);
Serial.println(irSensorValue);
In addition to the ultrasonic sensor, the IR sensor is also checked to determine whether an object is nearby. The IR sensor returns either a HIGH or LOW value, indicating the presence of an object (LOW) or its absence (HIGH). Like the distance, the sensor value is printed to the serial monitor for debugging.
Opening the Dustbin Lid
if (distance < 40 || irSensorValue == LOW) {
if (!lidOpen) {
lidOpen = true;
digitalWrite(ledPin, HIGH);
digitalWrite(lightPin, HIGH);
Serial.println("Led On");
for (int angle = 90; angle <= 180; angle++) {
servo1.write(angle);
servo2.write(180 - angle);
delay(10);
}
}
lastObjectTime = millis();
}
When an object is detected within 40 cm by the ultrasonic sensor or the IR sensor detects an object, the code checks if the lid is already open. If the lid is closed (lidOpen == false), it opens the lid by moving the servos in opposite directions. The servos gradually rotate from 90 degrees to 180 degrees to create a smooth opening motion. Meanwhile, the LED and light indicators turn on to provide visual feedback.
After the lid opens, the current time is recorded using millis(), which tracks the time when the object was last detected. This timestamp will be used later to determine when to close the lid.
Closing the Dustbin Lid and arduino uno spec
else {
if (lidOpen && millis() - lastObjectTime >= 2000) {
for (int angle = 180; angle >= 90; angle--) {
servo1.write(angle);
servo2.write(180 - angle);
delay(20);
}
lidOpen = false;
digitalWrite(ledPin, LOW);
digitalWrite(lightPin, LOW);
Serial.println("Led Off");
}
}
}
The Arduino then checks whether the lid has been open for more than 2 seconds, unless an object is detected or moves out of range. If this condition is met then servos trigger to rotate back to the original position (90 degrees) to gradually close the lid. Once the lid is fully closed, the LED and light indicators are disabled and the lid status is updated (lidOpen = false).
Summary
The Arduino based smart dustbin project uses both an ultrasonic as well as an IR sensor to find objects within range. The servos rotate the lid open and an LED lights up when an object is detected letting you know the dustbin is active. The lid automatically closes 2 seconds after the object moves away. The sensors take care of the dustbin opening only when needed, and the servo motors make the lid work smoothly. In this sense, the waste collection is efficient and friendly. For home automation enthusiasts wanting to make everyday items smart, this project will be ideal.
Conclusion: Impact and Future Potential
The power of automation in matters of cleanliness cannot be underscored enough, and using Arduino Nano, the Smart Dustbin shows us how simple it can be. I have developed this project by integrating sensors and microcontrollers with Arduino Uno specs and equivalent platforms to demonstrate how they can be used in creating practical solutions for the waste management problem. Moreover, this design can be extended further to include other features, for instance, Bluetooth or Wi-Fi connection for monitoring dustbin levels and as such make it an ideal design for smart city applications.
With focus on hands free operation, and use of reliable components like Arduino Nano and sensors, this project meets the current need of efficient and eco friendly method of handling waste and ensures a clean environment. The project is a stepping ground for building more sophisticated automation systems across different domains such as public sanitation, healthcare and industrial automation.